Given the
root
of a binary tree, return the level order traversal of its nodes’ values. (i.e., from left to right, level by level).Example 1:
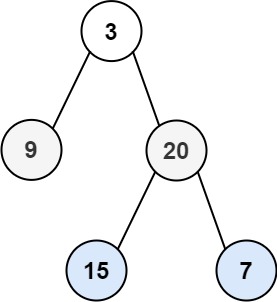
Input: root = [3,9,20,null,null,15,7]
Output: [[3],[9,20],[15,7]]
Example 2:
Input: root = [1]
Output: [[1]]
Example 3:
Input: root = []
Output: []
Constraints:
The number of nodes in the tree is in the range
[0, 2000]
.
-1000 <= Node.val <= 1000
题目大意:
二叉树按层遍历
解题思路:
用BFS模板
解题步骤:
N/A
注意事项:
- res.append(level)不是res.append(list(level))因为level = []已重新初始化。
- 关键行: 多这一行level.append(node.val),其他一样
Python代码:
1 | def levelOrder(self, root: TreeNode) -> List[List[int]]: |
算法分析:
时间复杂度为O(n)
,空间复杂度O(1)