Convert a Binary Search Tree to a sorted Circular Doubly-Linked List in place.
You can think of the left and right pointers as synonymous to the predecessor and successor pointers in a doubly-linked list. For a circular doubly linked list, the predecessor of the first element is the last element, and the successor of the last element is the first element.
We want to do the transformation in place. After the transformation, the left pointer of the tree node should point to its predecessor, and the right pointer should point to its successor. You should return the pointer to the smallest element of the linked list.
Example 1:
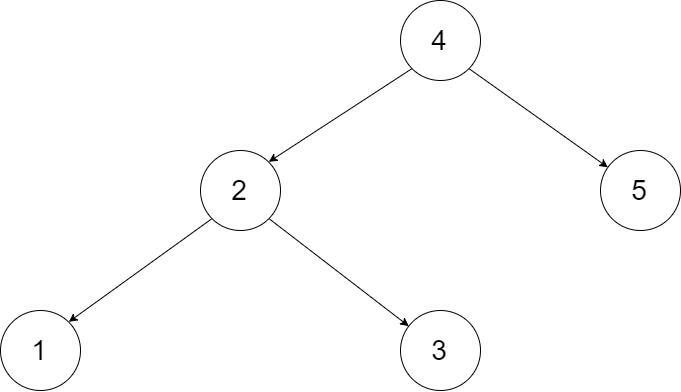
Input: root = [4,2,5,1,3]
Output: [1,2,3,4,5]
Explanation: The figure below shows the transformed BST. The solid line indicates the successor relationship, while the dashed line means the predecessor relationship.
Example 2:
Input: root = [2,1,3]
Output: [1,2,3]
Constraints:
The number of nodes in the tree is in the range
[0, 2000]
.
-1000 <= Node.val <= 1000
All the values of the tree are *unique.
题目大意:
将二叉树变成双向链表,left为父节点,right为儿子节点
解题思路:
类似于LeetCode 114 Flatten Binary Tree to Linked List,先假设左右儿子,已经是双向LL,下面就是将root这个节点插入到两个LL之间其将它们首尾相连
解题步骤:
N/A
注意事项:
- 要将左右儿子节点的LL,首尾相连。首尾节点获得要在if语句前,因为右儿子的left会连到root,就找不到它的尾部。首尾相连要发生在最后。
Python代码:
1 | def treeToDoublyList(self, root: 'Node') -> 'Node': |
算法分析:
时间复杂度为O(n)
,空间复杂度O(1)