Given a
root
of an N-ary tree, you need to compute the length of the diameter of the tree.The diameter of an N-ary tree is the length of the longest path between any two nodes in the tree. This path may or may not pass through the root.
(Nary-Tree input serialization is represented in their level order traversal, each group of children is separated by the null value.)
Example 1:
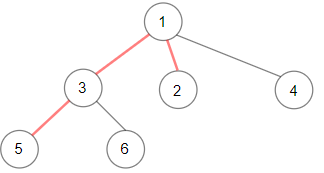
Input: root = [1,null,3,2,4,null,5,6]
Output: 3
Explanation: Diameter is shown in red color.
Example 2:
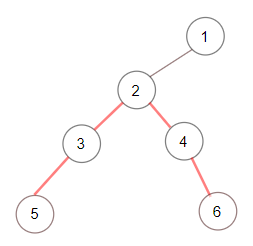
Input: root = [1,null,2,null,3,4,null,5,null,6]
Output: 4
Example 3:
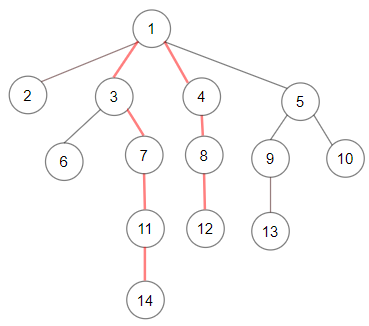
Input: root = [1,null,2,3,4,5,null,null,6,7,null,8,null,9,10,null,null,11,null,12,null,13,null,null,14]
Output: 7
Constraints:
The depth of the n-ary tree is less than or equal to
1000
.
The total number of nodes is between [1, 10<sup>4</sup>]
.题目大意:
求树的直径:任何两个节点的最大距离
解题思路:
类似于LeetCode 543 Diameter of Binary Tree,但此题为N叉树
解题步骤:
DFS
注意事项:
- 求数组中最大的两数和,用去掉最大值的方法得到次大值。还要注意初始值加入[1, 1],避免没有儿子节点或只有一个的情况
Python代码:
1 | def diameter(self, root: 'Node') -> int: |
算法分析:
时间复杂度为O(n)
,空间复杂度O(n)