Given the
root
of a binary tree, collect a tree’s nodes as if you were doing this:Collect all the leaf nodes. Remove all the leaf nodes.
Repeat until the tree is empty.
Example 1:
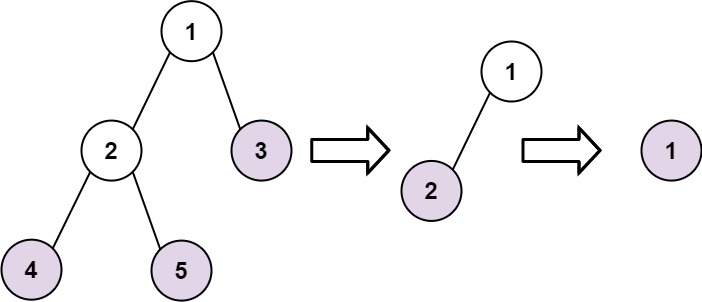
Input: root = [1,2,3,4,5]
Output: [[4,5,3],[2],[1]]
Explanation:
[[3,5,4],[2],[1]] and [[3,4,5],[2],[1]] are also considered correct answers since per each level it does not matter the order on which elements are returned.
Example 2:
Input: root = [1]
Output: [[1]]
Constraints: The number of nodes in the tree is in the range
[1, 100]
.*
-100 <= Node.val <= 100
题目大意:
求逐层叶子剥离的所有叶子节点,按剥离顺序放入结果
解题思路:
考虑BFS从上到下,但深度不对,因为是从叶子节点开始计算的,如例子所示,根节点1的高度取决于儿子的最大深度。所以应该从底到上计算,也就是DFS
解题步骤:
N/A
注意事项:
- 从底到上计算高度,取左右树的最大高度
Python代码:
1 | def findLeaves(self, root: TreeNode) -> List[List[int]]: |
算法分析:
时间复杂度为O(n)
,空间复杂度O(1)