Given an
m x n
integer matrix matrix
, if an element is 0
, set its entire row and column to 0
‘s, and return the matrix.You must do it in place.
Example 1:
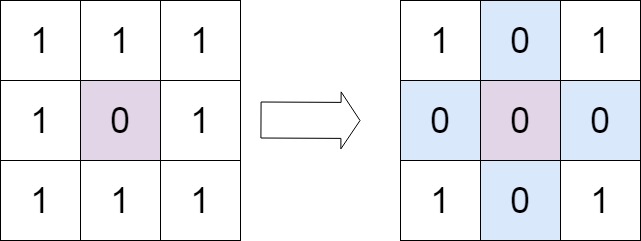
Input: matrix = [[1,1,1],[1,0,1],[1,1,1]]
Output: [[1,0,1],[0,0,0],[1,0,1]]
Example 2:
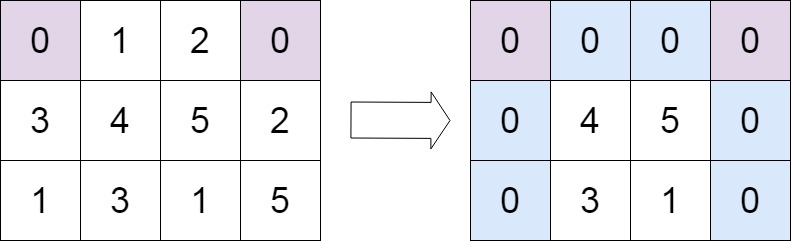
Input: matrix = [[0,1,2,0],[3,4,5,2],[1,3,1,5]]
Output: [[0,0,0,0],[0,4,5,0],[0,3,1,0]]
Constraints:
m == matrix.length
n == matrix[0].length
1 <= m, n <= 200
-2<sup>31</sup> <= matrix[i][j] <= 2<sup>31</sup> - 1
Follow up:
A straightforward solution using
O(mn)
space is probably a bad idea.
A simple improvement uses O(m + n)
space, but still not the best solution.* Could you devise a constant space solution?
题目大意:
若矩阵某元素为0,设置它所在的行和列所有元素均为0,不能用额外区间
解题思路:
用第0行和第0列作为统计。由于第0行和第0列会被覆盖,所以先查看他们有无0
解题步骤:
N/A
注意事项:
- 用第0行和第0列作为统计。由于第0行和第0列会被覆盖,所以先查看他们有无0。两大步骤:先统计,再根据结果设置0
- 第二步中,根据第0和和第0列的结果回设,均从1开始,不含左上cell,因为统计结果不保存在它上。它仅在统计第一行和第一列是否有0时用到。
Python代码:
1 | def setZeroes(self, matrix: List[List[int]]) -> None: |
算法分析:
时间复杂度为O(n2)
,空间复杂度O(1)