You are given an
n x n
2D matrix
representing an image, rotate the image by 90 degrees (clockwise).You have to rotate the image in-place, which means you have to modify the input 2D matrix directly. DO NOT allocate another 2D matrix and do the rotation.
Example 1:
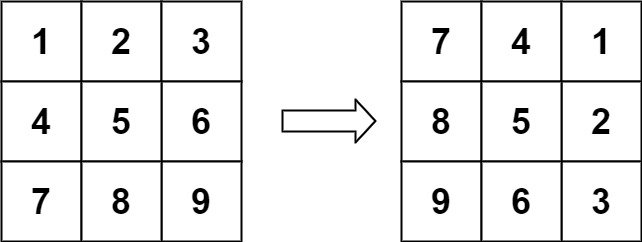
Input: matrix = [[1,2,3],[4,5,6],[7,8,9]]
Output: [[7,4,1],[8,5,2],[9,6,3]]
Example 2:
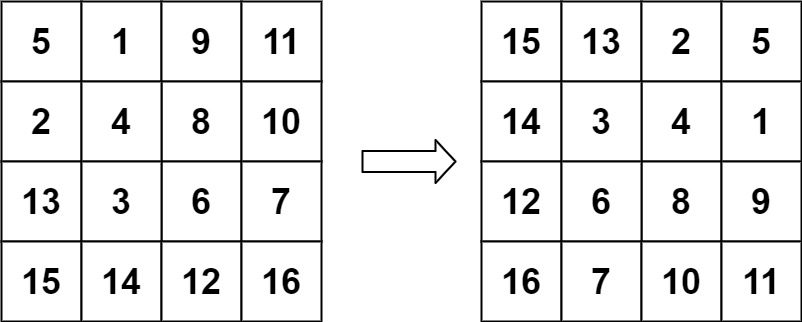
Input: matrix = [[5,1,9,11],[2,4,8,10],[13,3,6,7],[15,14,12,16]]
Output: [[15,13,2,5],[14,3,4,1],[12,6,8,9],[16,7,10,11]]
Constraints:
n == matrix.length == matrix[i].length
1 <= n <= 20
*
-1000 <= matrix[i][j] <= 1000
题目大意:
顺时针循环矩阵90度
解题思路:
先上下对称,再沿正对角线(左上到右下)对称。正对角线实现比较容易
解题步骤:
N/A
注意事项:
- 先上下对称,再沿正对角线(左上到右下)对称。正对角线实现比较容易
Python代码:
1 | def rotate(self, matrix: List[List[int]]) -> None: |
算法分析:
时间复杂度为O(n2)
,空间复杂度O(1)