Given the
root
of a binary tree, return the zigzag level order traversal of its nodes’ values. (i.e., from left to right, then right to left for the next level and alternate between).Example 1:
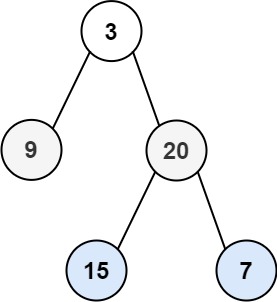
Input: root = [3,9,20,null,null,15,7]
Output: [[3],[20,9],[15,7]]
Example 2:
Input: root = [1]
Output: [[1]]
Example 3:
Input: root = []
Output: []
Constraints:
The number of nodes in the tree is in the range
[0, 2000]
.
-100 <= Node.val <= 100
题目大意:
按层遍历二叉树。偶数层逆向
解题思路:
用BFS按层遍历模板
解题步骤:
N/A
注意事项:
- 多这一行level.append(node.val)
Python代码:
1 | def zigzagLevelOrder(self, root: TreeNode) -> List[List[int]]: |
算法分析:
时间复杂度为O(n)
,空间复杂度O(1)