Given an array of
points
where points[i] = [x<sub>i</sub>, y<sub>i</sub>]
represents a point on the X-Y plane and an integer k
, return the k
closest points to the origin (0, 0)
.The distance between two points on the X-Y plane is the Euclidean distance (i.e.,
√(x<sub>1</sub> - x<sub>2</sub>)<sup>2</sup> + (y<sub>1</sub> - y<sub>2</sub>)<sup>2</sup>
).You may return the answer in any order. The answer is guaranteed to be unique (except for the order that it is in).
Example 1:
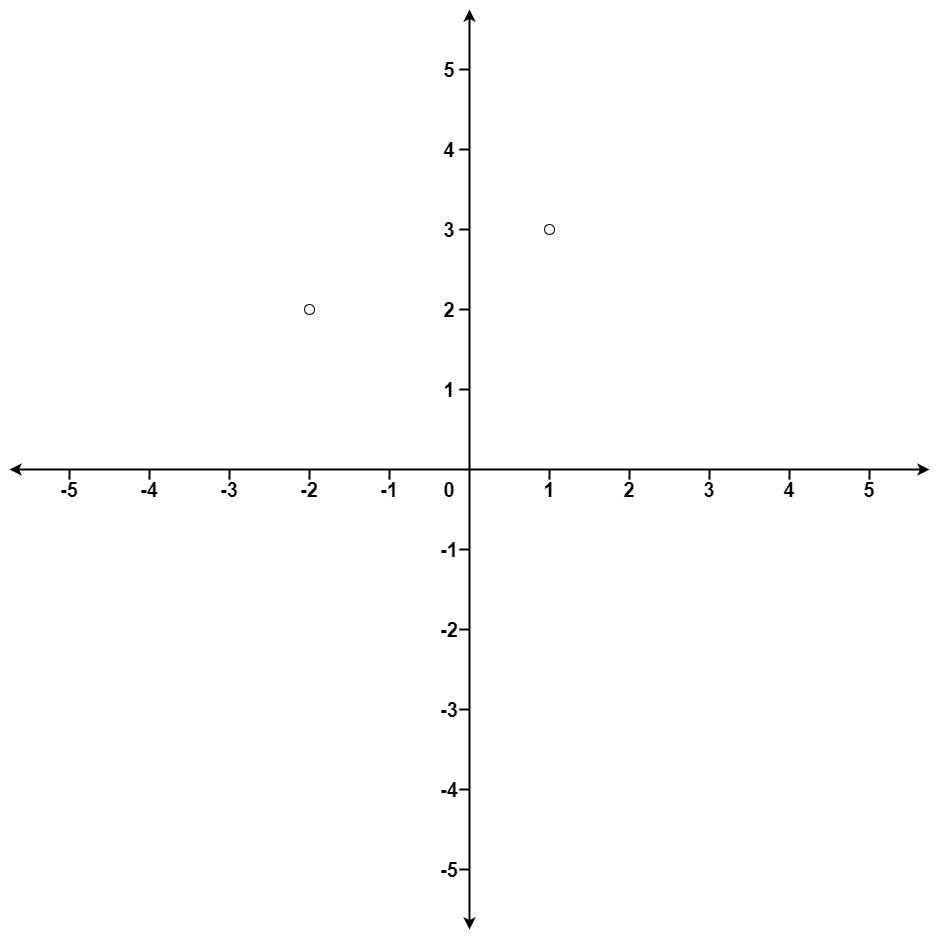
Input: points = [[1,3],[-2,2]], k = 1
Output: [[-2,2]]
Explanation:
The distance between (1, 3) and the origin is sqrt(10).
The distance between (-2, 2) and the origin is sqrt(8).
Since sqrt(8) < sqrt(10), (-2, 2) is closer to the origin.
We only want the closest k = 1 points from the origin, so the answer is just [[-2,2]].
Example 2:
Input: points = [[3,3],[5,-1],[-2,4]], k = 2
Output: [[3,3],[-2,4]]
Explanation: The answer [[-2,4],[3,3]] would also be accepted.
Constraints:
1 <= k <= points.length <= 10<sup>4</sup>
-10<sup>4</sup> < x<sub>i</sub>, y<sub>i</sub> < 10<sup>4</sup>
算法思路:
最大堆
注意事项:
- 求最小距离用最大堆,距离的相反数入堆
- 与堆顶比较,跟模板一样仍然是大于号
Python代码:
1 | def kClosest(self, points: List[List[int]], k: int) -> List[List[int]]: |
Java代码:
1 | public int[][] kClosest(int[][] points, int K) { |
算法分析:
时间复杂度为O(nlogk)
,空间复杂度O(k)