Given two integer arrays
preorder
and inorder
where preorder
is the preorder traversal of a binary tree and inorder
is the inorder traversal of the same tree, construct and return the binary tree.Example 1:
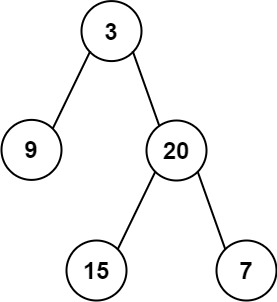
Input: preorder = [3,9,20,15,7], inorder = [9,3,15,20,7]
Output: [3,9,20,null,null,15,7]
Example 2:
Input: preorder = [-1], inorder = [-1]
Output: [-1]
Constraints:
1 <= preorder.length <= 3000
inorder.length == preorder.length
-3000 <= preorder[i], inorder[i] <= 3000
preorder
and inorder
consist of unique values.Each value of
inorder
also appears in preorder
.
preorder
is guaranteed to be the preorder traversal of the tree.inorder
is *guaranteed to be the inorder traversal of the tree.算法思路:
N/A
注意事项:
- 用递归实现,in_order字符串分左右两段子串递归到左右儿子,pre_order字符串每轮递归用pop(0)原地去除首位,再递归到儿子节点
- 终止条件为in_order字符串为空。
Python代码:
1 | def buildTree(self, preorder: List[int], inorder: List[int]) -> TreeNode: |
算法分析:
时间复杂度为O(n)
,空间复杂度O(1)