Given the
root
of a binary tree, determine if it is a valid binary search tree (BST).A valid BST is defined as follows:
The left subtree of a node contains only nodes with keys less than the node’s key. The right subtree of a node contains only nodes with keys greater than the node’s key.
Both the left and right subtrees must also be binary search trees.
Example 1:
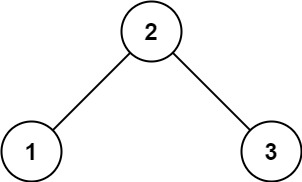
Input: root = [2,1,3]
Output: true
Example 2:
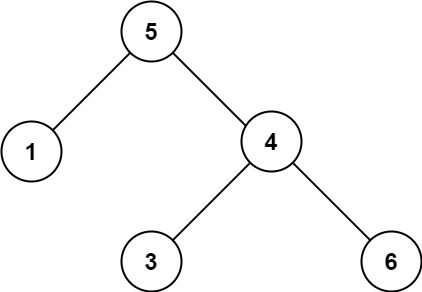
Input: root = [5,1,4,null,null,3,6]
Output: false
Explanation: The root node’s value is 5 but its right child’s value is 4.
Constraints: The number of nodes in the tree is in the range
[1, 10<sup>4</sup>]
.*
-2<sup>31</sup> <= Node.val <= 2<sup>31</sup> - 1
题目大意:
验证BST
算法思路:
N/A
注意事项:
- 用min, max法
Python代码:
1 | def isValidBST(self, root: TreeNode) -> bool: |
Java代码:
1 | // Recommended method: use min max and devide & conquer |
算法分析:
时间复杂度为O(n)
,空间复杂度O(1)