Given a
n * n
matrix grid
of 0's
and 1's
only. We want to represent the grid
with a Quad-Tree.Return the root of the Quad-Tree representing the
grid
.Notice that you can assign the value of a node to True or False when
isLeaf
is False, and both are accepted in the answer.A Quad-Tree is a tree data structure in which each internal node has exactly four children. Besides, each node has two attributes:
val
: True if the node represents a grid of 1’s or False if the node represents a grid of 0’s.
isLeaf
: True if the node is leaf node on the tree or False if the node has the four children.class Node {
public boolean val;
public boolean isLeaf;
public Node topLeft;
public Node topRight;
public Node bottomLeft;
public Node bottomRight;
}
We can construct a Quad-Tree from a two-dimensional area using the following steps:
1. If the current grid has the same value (i.e all
1's
or all 0's
) set isLeaf
True and set val
to the value of the grid and set the four children to Null and stop.2. If the current grid has different values, set
isLeaf
to False and set val
to any value and divide the current grid into four sub-grids as shown in the photo.3. Recurse for each of the children with the proper sub-grid.
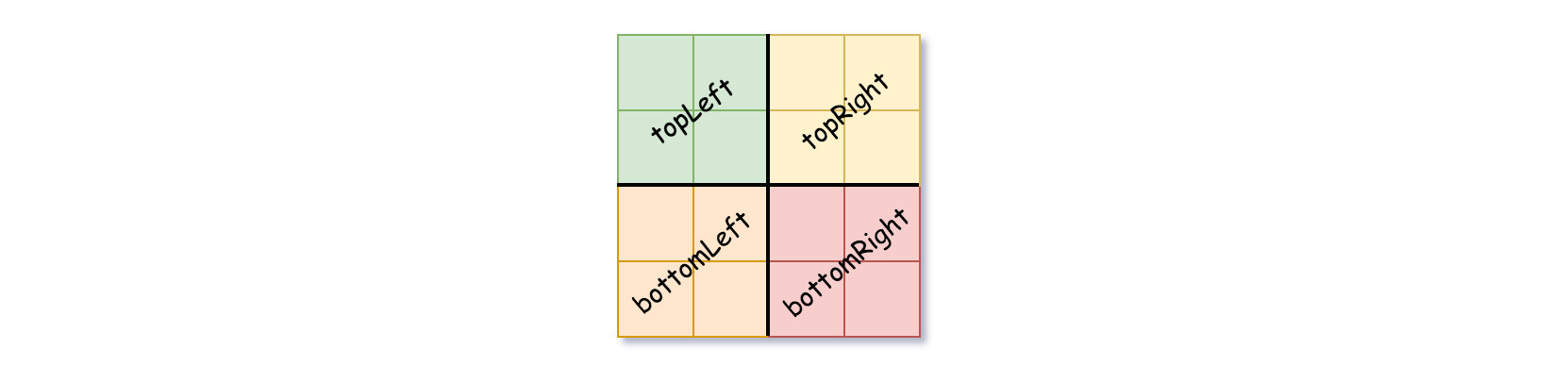
If you want to know more about the Quad-Tree, you can refer to the wiki.
Quad-Tree format:
The output represents the serialized format of a Quad-Tree using level order traversal, where
null
signifies a path terminator where no node exists below.It is very similar to the serialization of the binary tree. The only difference is that the node is represented as a list
[isLeaf, val]
.If the value of
isLeaf
or val
is True we represent it as 1 in the list [isLeaf, val]
and if the value of isLeaf
or val
is False we represent it as 0.Example 1:

Input: grid = [[0,1],[1,0]]
Output: [[0,1],[1,0],[1,1],[1,1],[1,0]]
Explanation: The explanation of this example is shown below:
Notice that 0 represnts False and 1 represents True in the photo representing the Quad-Tree.
Example 2:
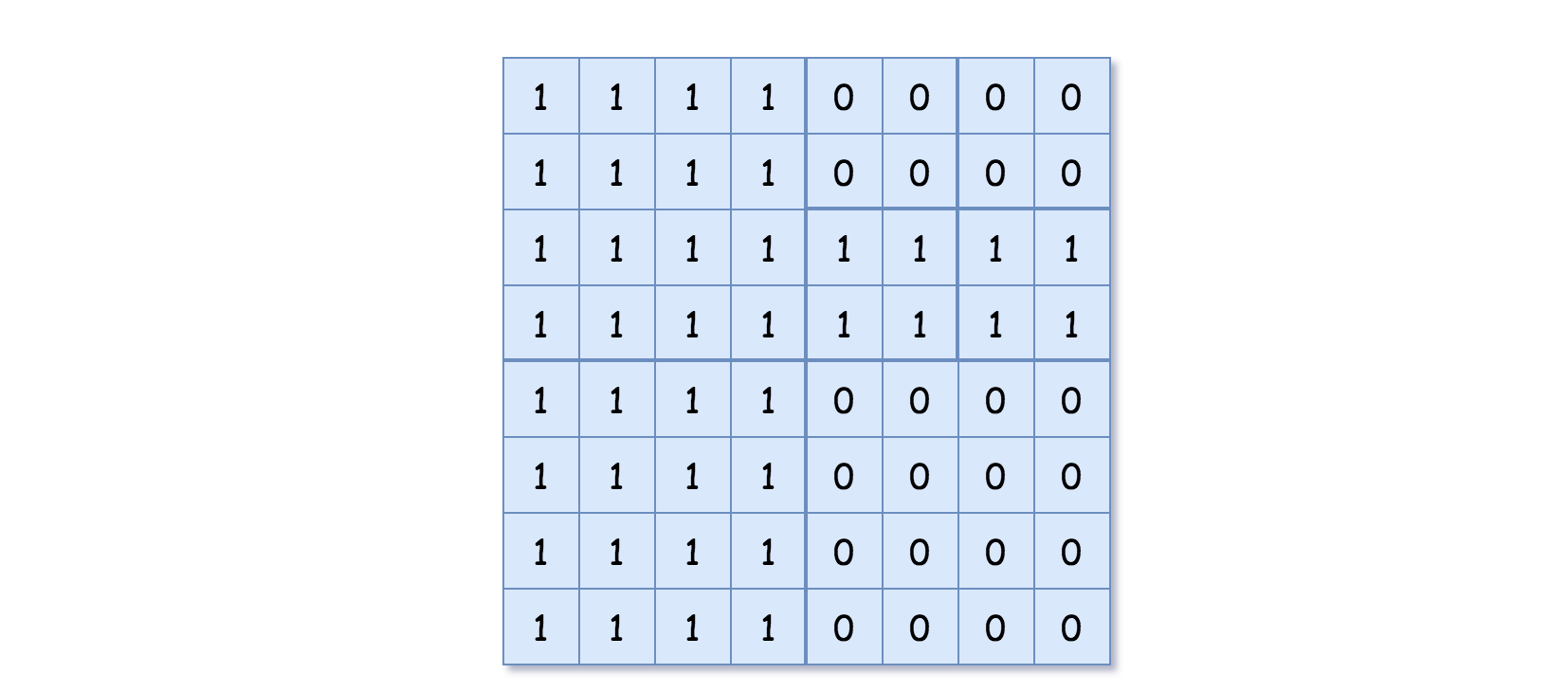
Input: grid = [[1,1,1,1,0,0,0,0],[1,1,1,1,0,0,0,0],[1,1,1,1,1,1,1,1],[1,1,1,1,1,1,1,1],[1,1,1,1,0,0,0,0],[1,1,1,1,0,0,0,0],[1,1,1,1,0,0,0,0],[1,1,1,1,0,0,0,0]]
Output: [[0,1],[1,1],[0,1],[1,1],[1,0],null,null,null,null,[1,0],[1,0],[1,1],[1,1]]
Explanation: All values in the grid are not the same. We divide the grid into four sub-grids.
The topLeft, bottomLeft and bottomRight each has the same value.
The topRight have different values so we divide it into 4 sub-grids where each has the same value.
Explanation is shown in the photo below:
Constraints:
n == grid.length == grid[i].length
n == 2<sup>x</sup>
where 0 <= x <= 6
题目大意:
由矩阵建四叉树。矩阵有0和1组成。按以下步骤:若子矩阵(变成为2的幂)只含1或0,生成一个叶子节点,值为该值;子矩阵含0和1混合,值为0或1(均为答案),非叶子节点,递归四个同样大小的矩阵生成相应节点。
矩阵大小为2的幂,最小长度为1.
单格DFS算法II解题思路(推荐):
也是DFS,但递归终止条件为长度1,也就是每个cell都是叶子节点,先递归然后再归纳,若四个儿子节点都是叶子节点且值都相等,合并为一个叶子节点。否则为非叶子节点。
此算法实现更简单,但比较难想出。上述方法思想是按照题意。
注意事项:
- size = 1作为终止条件
- 两个条件该轮递归的节点为叶子节点,第一值相等,第二儿子节点都是叶子节点
Python代码:
1 | def construct(self, grid: List[List[int]]) -> 'Node': |
算法分析:
时间复杂度为O(n2)
,空间复杂度
O(1)`
presum解题思路(不推荐):
这是我的方法,按照定义求解,定义是递归的,所以用DFS。而统计子矩阵和用presum提高效率。但实现比较复杂
解题步骤:
N/A
注意事项:
- 子矩阵presum用模板
- 终止条件为子矩阵sum是0或n平方
Python代码:
1 | def construct(self, grid: List[List[int]]) -> 'Node': |
算法分析:
时间复杂度为O(n2)
`,空间复杂度O(n2)