You are given an
m x n
binary matrix grid
.In one operation, you can choose any row or column and flip each value in that row or column (i.e., changing all
0
‘s to 1
‘s, and all 1
‘s to 0
‘s).Return
true
if it is possible to remove all 1
‘s from grid
using any number of operations or false
otherwise.Example 1:
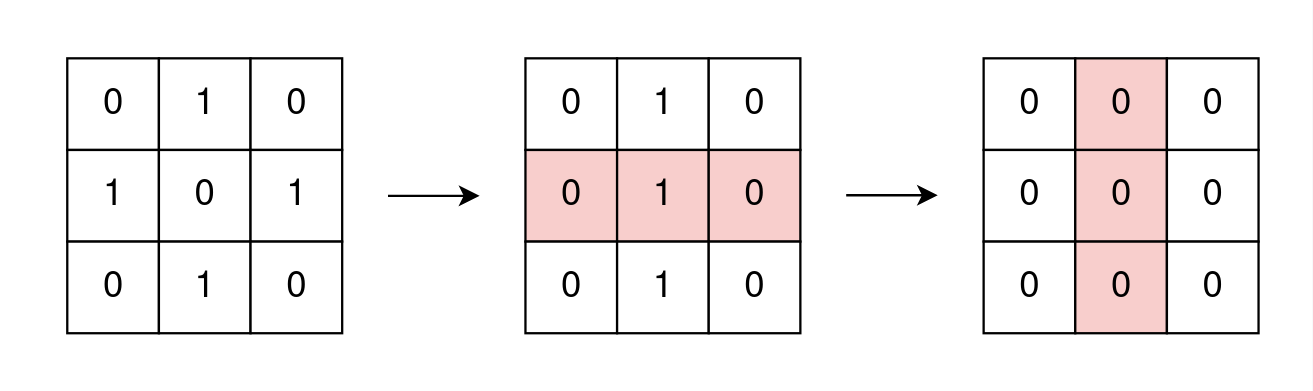
Input: grid = [[0,1,0],[1,0,1],[0,1,0]]
Output: true
Explanation: One possible way to remove all 1’s from grid is to:
- Flip the middle row
- Flip the middle column
Example 2:
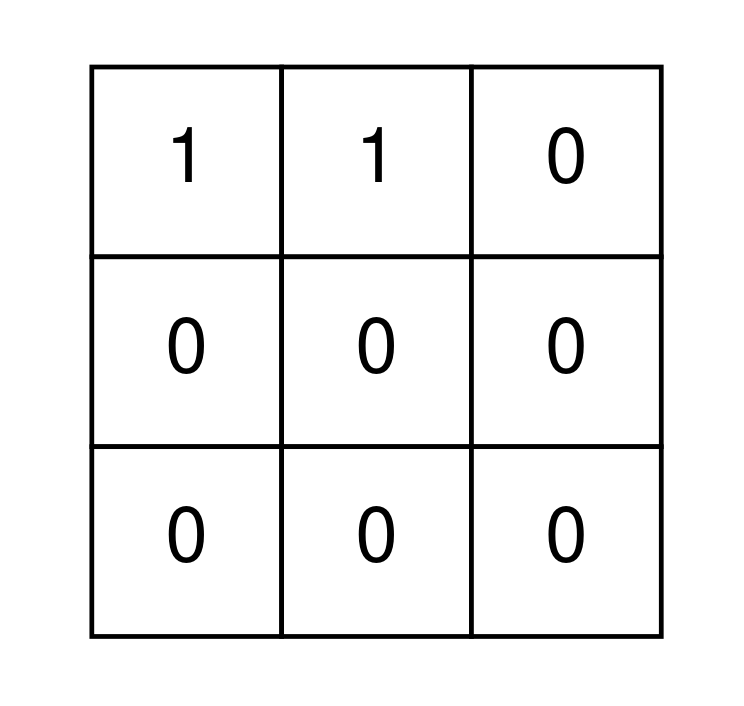
Input: grid = [[1,1,0],[0,0,0],[0,0,0]]
Output: false
Explanation: It is impossible to remove all 1’s from grid.
Example 3:
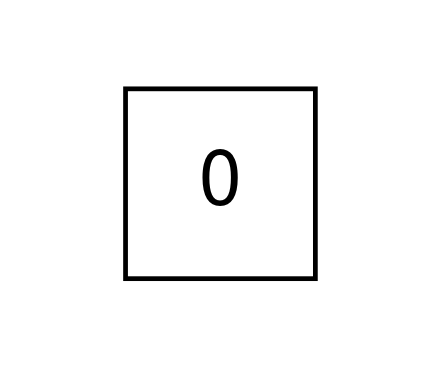
Input: grid = [[0]]
Output: true
Explanation: There are no 1’s in grid.
Constraints:
m == grid.length
n == grid[i].length
1 <= m, n <= 300
grid[i][j]
is either 0
or 1
.题目大意:
给定一个矩阵,每次可以flip一行或一列,求是否可以令矩阵变成全0
解题思路:
从例子找规律,
010和010属于一种类型
010和101也是同一种,每一行必须符合任何一种类型才是解
解题步骤:
N/A
注意事项:
Python代码:
1
2
3
4
5
6def removeOnes(self, grid: List[List[int]]) -> bool:
row_patten, row_pattern_invert = grid[0], [1 - n for n in grid[0]]
for i in range(1, len(grid)):
if grid[i] != row_patten and grid[i] != row_pattern_invert:
return False
return True
算法分析:
时间复杂度为O(n2)
,空间复杂度O(n)