The n-queens puzzle is the problem of placing n
queens on an n x n
chessboard such that no two queens attack each other.
Given an integer n
, return all distinct solutions to the n-queens puzzle. You may return the answer in any order.
Each solution contains a distinct board configuration of the n-queens’ placement, where ‘Q’
and ‘.’
both indicate a queen and an empty space, respectively.
Example 1:
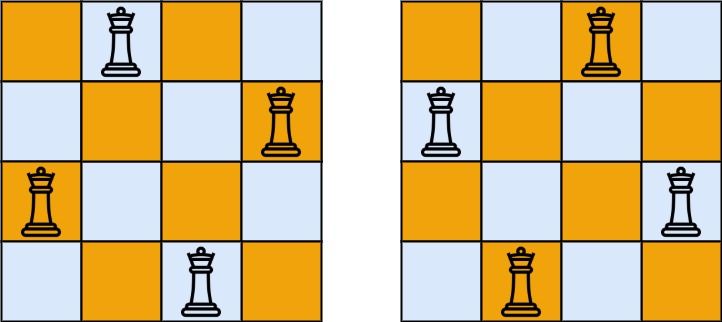
Input: n = 4
Output: [[“.Q..”,”…Q”,”Q…”,”..Q.”],[“..Q.”,”Q…”,”…Q”,”.Q..”]]
Explanation: There exist two distinct solutions to the 4-queens puzzle as shown above
Example 2:
Input: n = 1
Output: [[“Q”]]
Constraints:
1 <= n <= 9
题目大意:
八皇后问题,求所有解
Global dict算法思路(推荐):
DFS填位法模板
注意事项:
- 用3个set,col_set, 对角线,反对角线set来提高效率。
- path用append的方法加入,这样终止条件用n来比较,不能用len(path)
- 打印函数中,one_result在每轮后reset;字符串不能改其中一个,只能用子串+Q+子串: (‘.’ path[i]) + ‘Q’ + (‘.’ (n - path[i] - 1))
Python代码:
1 | def solveNQueens(self, n: int) -> List[List[str]]: |
算法分析:
时间复杂度为O(n!)
,空间复杂度O(n^2)
常数空间算法II解题思路(推荐):
较直观的方法,但复杂度稍差
Python代码:
1 | def solveNQueens2(self, n: int) -> List[List[str]]: |
Java代码:
1 | public List<List<String>> solveNQueens2(int n) { |
算法分析:
时间复杂度为O(n!)
,空间复杂度O(n^2)