LeetCode 173 Binary Search Tree Iterator
Implement an iterator over a binary search tree (BST). Your iterator will be initialized with the root node of a BST.
Calling
next()
will return the next smallest number in the BST.Example:
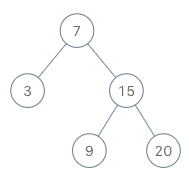
BSTIterator iterator = new BSTIterator(root);
iterator.next(); // return 3
iterator.next(); // return 7
iterator.hasNext(); // return true
iterator.next(); // return 9
iterator.hasNext(); // return true
iterator.next(); // return 15
iterator.hasNext(); // return true
iterator.next(); // return 20
iterator.hasNext(); // return false
Note:
next()
and hasNext()
should run in average O(1) time and uses O(h) memory, where h is the height of the tree.
You may assume that next()
call will always be valid, that is, there will be at least a next smallest number in the BST when next()
is called.题目大意:
实现BST的Iterator
算法思路:
参照KB中BST的非递归中序遍历。将其分拆为初始化以及去掉stack不为空的循环分别为所求。
注意事项:
- 初始化,将root所有左节点加入到stack。先写next,出栈栈顶节点,将它的右儿子的所有左节点入栈。
Python代码:
1 | class BSTIterator(TestCases): |
Java代码:
1 | Stack<TreeNode> s = new Stack<>(); |
算法分析:
next的平均时间复杂度(amortized complexity)为O(1)
,n为字符串长度,空间复杂度O(logn)
。